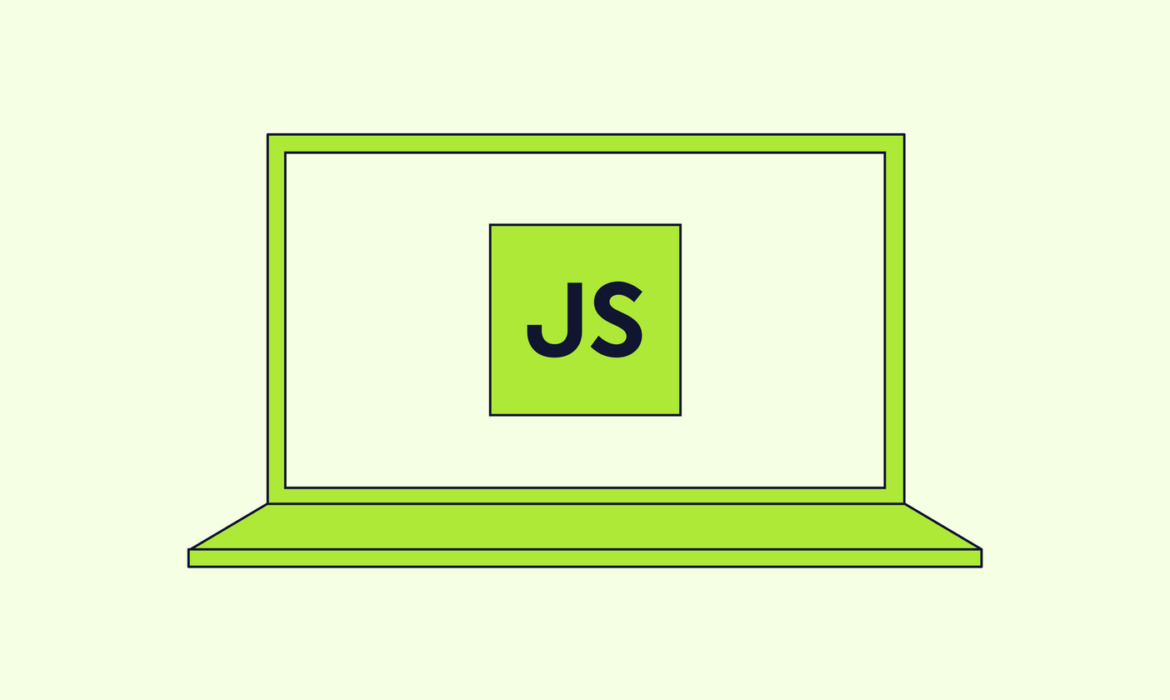
JavaScript is the backbone of web development, enabling interactive and dynamic content on websites. Whether you’re new to programming or brushing up on your skills, grasping the core functionalities of JavaScript is essential for creating engaging and efficient web applications. In this blog, we’ll explore the fundamental aspects of JavaScript that form the foundation of effective coding.
1. Variables and Data Types
JavaScript uses variables to store data that can be referenced and manipulated. Understanding data types is crucial for effective programming.
- Variables: Declared using
var
,let
, orconst
. The choice of keyword affects the variable’s scope and mutability.javascriptCopy codelet name = "John"; const age = 30; var isActive = true;
- Data Types: JavaScript has several basic data types:
- String: Represents text.javascriptCopy code
let message = "Hello, World!";
- Number: Represents both integer and floating-point numbers.javascriptCopy code
let count = 10; let price = 19.99;
- Boolean: Represents true or false values.javascriptCopy code
let isAvailable = false;
- Object: Represents collections of key-value pairs.javascriptCopy code
let person = { name: "Alice", age: 25 };
- Array: Represents ordered lists of values.javascriptCopy code
let fruits = ["apple", "banana", "cherry"];
- String: Represents text.javascriptCopy code
2. Operators
Operators are symbols used to perform operations on variables and values. JavaScript supports various types of operators:
- Arithmetic Operators: Perform basic math operations.javascriptCopy code
let sum = 5 + 3; // Addition let product = 4 * 2; // Multiplication
- Comparison Operators: Compare two values and return a boolean result.javascriptCopy code
let isEqual = (5 === 5); // Strict equality let isGreater = (10 > 5); // Greater than
- Logical Operators: Combine boolean values.javascriptCopy code
let andCondition = (true && false); // Logical AND let orCondition = (true || false); // Logical OR
- Assignment Operators: Assign values to variables.javascriptCopy code
let x = 10; x += 5; // Equivalent to x = x + 5
3. Control Flow
Control flow statements manage the direction of code execution based on conditions.
- Conditional Statements: Execute code based on conditions.javascriptCopy code
let age = 18; if (age >= 18) { console.log("Adult"); } else { console.log("Minor"); }
- Switch Statement: Select one of many code blocks to execute.javascriptCopy code
let day = 3; switch (day) { case 1: console.log("Monday"); break; case 2: console.log("Tuesday"); break; default: console.log("Other day"); }
- Loops: Repeat code execution.
- for Loop: Iterates a specific number of times.javascriptCopy code
for (let i = 0; i < 5; i++) { console.log(i); }
- while Loop: Repeats as long as a condition is true.javascriptCopy code
let i = 0; while (i < 5) { console.log(i); i++; }
- for Loop: Iterates a specific number of times.javascriptCopy code
4. Functions
Functions are reusable blocks of code that perform specific tasks.
- Function Declaration: Defines a named function.javascriptCopy code
function greet(name) { return "Hello, " + name; }
- Function Expression: Defines a function as a value assigned to a variable.javascriptCopy code
let square = function(number) { return number * number; };
- Arrow Functions: Provide a concise syntax for function expressions.javascriptCopy code
let add = (a, b) => a + b;
5. Objects and Arrays
Objects and arrays are fundamental structures for storing and managing data.
- Objects: Collections of key-value pairs.javascriptCopy code
let car = { make: "Toyota", model: "Corolla", year: 2020 }; console.log(car.make); // Access property
- Arrays: Ordered collections of values.javascriptCopy code
let numbers = [1, 2, 3, 4, 5]; console.log(numbers[0]); // Access element
6. Events and DOM Manipulation
JavaScript can interact with the HTML Document Object Model (DOM) to create dynamic web pages.
- Event Handling: Respond to user actions like clicks or key presses.javascriptCopy code
document.getElementById("myButton").addEventListener("click", function() { alert("Button clicked!"); });
- DOM Manipulation: Change the content and structure of the web page.javascriptCopy code
document.getElementById("myDiv").innerHTML = "New content!";
7. Asynchronous JavaScript
JavaScript handles asynchronous operations to manage tasks like API calls or timers.
- Callbacks: Functions passed as arguments to be executed later.javascriptCopy code
function fetchData(callback) { setTimeout(() => { callback("Data received"); }, 2000); } fetchData(function(result) { console.log(result); });
- Promises: Represent the completion (or failure) of an asynchronous operation.javascriptCopy code
let promise = new Promise((resolve, reject) => { setTimeout(() => resolve("Data received"), 2000); }); promise.then(result => console.log(result));
- Async/Await: Syntactic sugar for working with promises, making asynchronous code easier to read.javascriptCopy code
async function fetchData() { let response = await new Promise((resolve) => { setTimeout(() => resolve("Data received"), 2000); }); console.log(response); } fetchData();
Conclusion
Understanding JavaScript’s basic functionality is essential for building dynamic and interactive web applications. From managing variables and operators to handling asynchronous tasks and manipulating the DOM, mastering these fundamentals will set the stage for more advanced programming concepts. Whether you’re just starting or looking to refresh your skills, a solid grasp of these core principles will empower you to create effective and engaging web experiences.