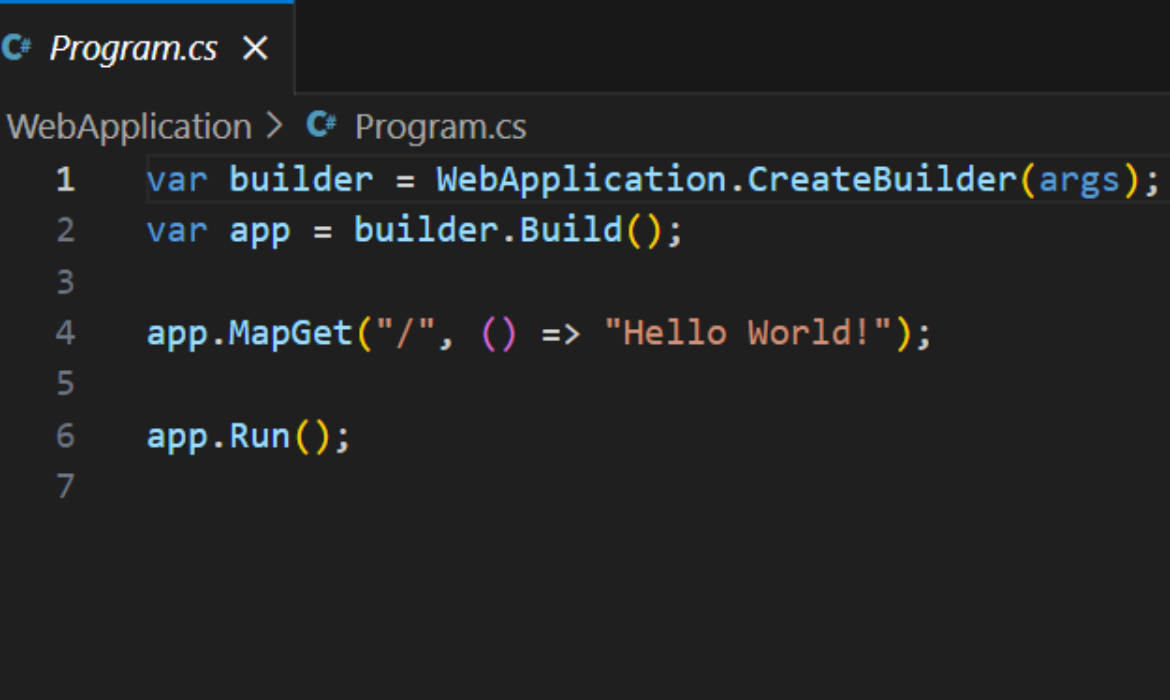
Why Does NullReferenceException
Happen?
In .NET, reference types can be null, meaning they don’t point to any object. When you try to call a method or access a property on a null reference, you get a NullReferenceException
. For example:
csharpCopy codestring myString = null;
int length = myString.Length; // This will throw a NullReferenceException
In this code, myString
is null
, so trying to access its Length
property causes an exception.
Common Scenarios Leading to NullReferenceException
- Uninitialized Variables:csharpCopy code
MyClass obj; obj.DoSomething(); // obj is not initialized
- Missing Object Creation:csharpCopy code
MyClass obj = null; obj.DoSomething(); // obj is explicitly set to null
- Dereferencing a Method Result:csharpCopy code
MyClass obj = GetObject(); obj.DoSomething(); // GetObject() might return null
- Chained Calls:csharpCopy code
MyClass obj = new MyClass(); obj.ChildObject.DoSomething(); // ChildObject might be null
How to Prevent NullReferenceException
- Initialize Variables: Always initialize your reference variables before using them.csharpCopy code
MyClass obj = new MyClass(); obj.DoSomething();
- Check for Null: Use null checks to ensure that your object is not null before accessing its members.csharpCopy code
if (myString != null) { int length = myString.Length; }
- Use Null-Conditional Operator: C# provides the null-conditional operator (
?.
) to simplify null checks.csharpCopy codeint? length = myString?.Length;
- Default Value with Null-Coalescing Operator: Use the null-coalescing operator (
??
) to provide a default value.csharpCopy codeint length = myString?.Length ?? 0;
- Nullable Reference Types: In C# 8.0 and later, you can enable nullable reference types to get compile-time warnings for potential null dereferences.csharpCopy code
string? myString = null; // The compiler will warn if you access myString without a null check
Example with Null-Conditional Operator
Here’s a practical example using the null-conditional operator to avoid NullReferenceException
:
csharpCopy codepublic class Person
{
public string Name { get; set; }
public Address Address { get; set; }
}
public class Address
{
public string City { get; set; }
}
public void PrintCity(Person person)
{
// Using null-conditional operator to safely access City property
string city = person?.Address?.City ?? "City not available";
Console.WriteLine(city);
}
In this example, the PrintCity
method safely accesses the City
property even if person
or person.Address
is null.
Conclusion
NullReferenceException
is a common but avoidable error in .NET development. By initializing variables, performing null checks, and using features like the null-conditional and null-coalescing operators, you can write more robust and error-free code. Adopting these practices will help you avoid runtime exceptions and make your applications more reliable.