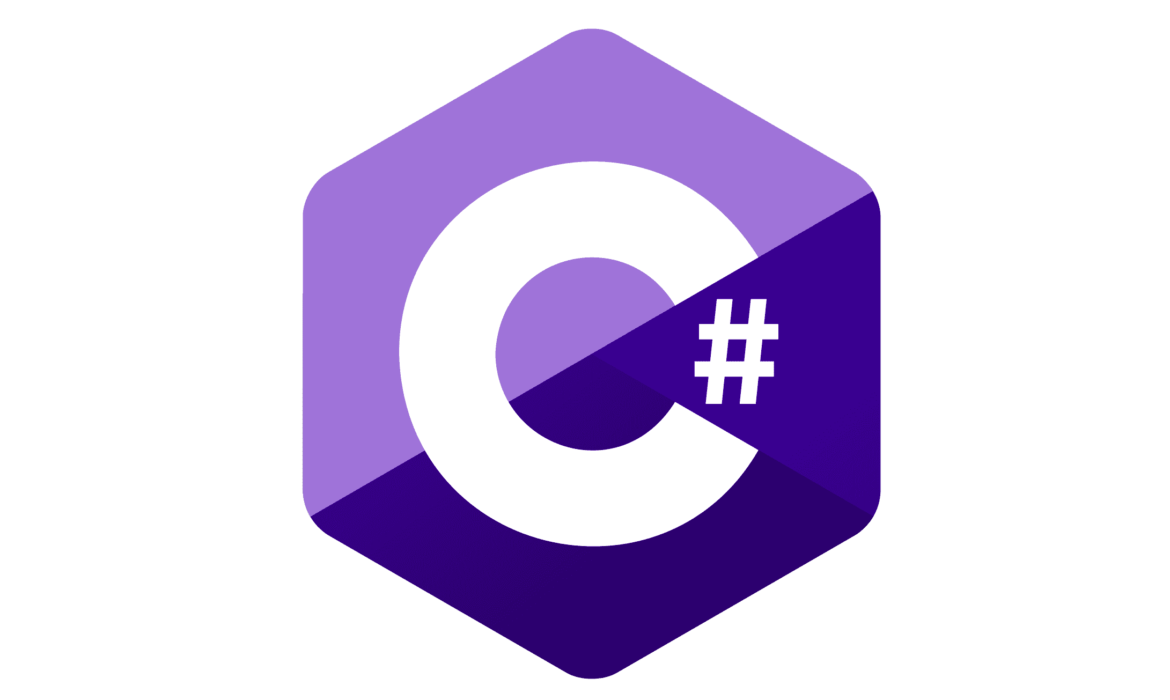
How to Automatically Set “From Date” to Yesterday and “To Date” to Today in ASP.NET C#
In ASP.NET web applications, date fields are often used in forms to allow users to input or filter data by specific time ranges. To make this process easier for users, it’s useful to automatically prepopulate these fields with logical defaults. For example, setting a “From Date” to yesterday and a “To Date” to today can be quite practical in scenarios such as generating daily reports or setting up search filters.
In this article, we will walk you through how to set these default dates in ASP.NET C# without using JavaScript.
Step-by-Step Implementation:
Step 1: HTML Setup with TextBox
Controls
First, create two TextBox
controls for the “From Date” and “To Date” in your ASP.NET web form. Here’s the HTML part where we will use the CalendarExtender
and MaskedEditExtender
controls to make sure the date input is user-friendly.
htmlCopy code<!-- From Date Field (Yesterday) -->
<label for="">दिनांक से (From Date) </label>
<asp:TextBox runat="server" ID="txtfromdate" class="form-control"></asp:TextBox>
<ajx:CalendarExtender ID="calendarFromDate" runat="server" TargetControlID="txtfromdate" Format="dd/MM/yyyy"></ajx:CalendarExtender>
<ajx:MaskedEditExtender ID="maskFromDate" runat="server" TargetControlID="txtfromdate" MaskType="Date" Mask="99/99/9999" />
<!-- To Date Field (Today) -->
<label for="">दिनांक तक (To Date) </label>
<asp:TextBox runat="server" ID="txttodate" class="form-control"></asp:TextBox>
<ajx:CalendarExtender ID="calendarToDate" runat="server" TargetControlID="txttodate" Format="dd/MM/yyyy"></ajx:CalendarExtender>
<ajx:MaskedEditExtender ID="maskToDate" runat="server" TargetControlID="txttodate" MaskType="Date" Mask="99/99/9999" />
In the above markup, we are using ASP.NET’s AJAX Calendar Extender and MaskedEditExtender to allow users to select and format dates easily.
Step 2: C# Code-Behind Logic for Setting Default Dates
Now, in the Page_Load
method of your code-behind (C# file), we’ll write the logic to automatically set the “From Date” to yesterday and the “To Date” to today’s date.
csharpCopy codeprotected void Page_Load(object sender, EventArgs e)
{
// Ensure that the code runs only when the page is first loaded
if (!IsPostBack)
{
// Set 'From Date' to Yesterday
txtfromdate.Text = DateTime.Now.AddDays(-1).ToString("dd/MM/yyyy");
// Set 'To Date' to Today
txttodate.Text = DateTime.Now.ToString("dd/MM/yyyy");
}
}
Step 3: Explanation of Code
if (!IsPostBack)
: This ensures that the dates are set only when the page is loaded for the first time and not during subsequent postbacks.DateTime.Now.AddDays(-1)
: This method subtracts one day from the current date, effectively setting the “From Date” to yesterday.DateTime.Now.ToString("dd/MM/yyyy")
: This formats the current date to the “dd/MM/yyyy” format and assigns it to the “To Date” field.
With these steps, the dates will automatically appear in the input fields when the page loads. This saves time for users and avoids unnecessary clicks to select the dates manually.