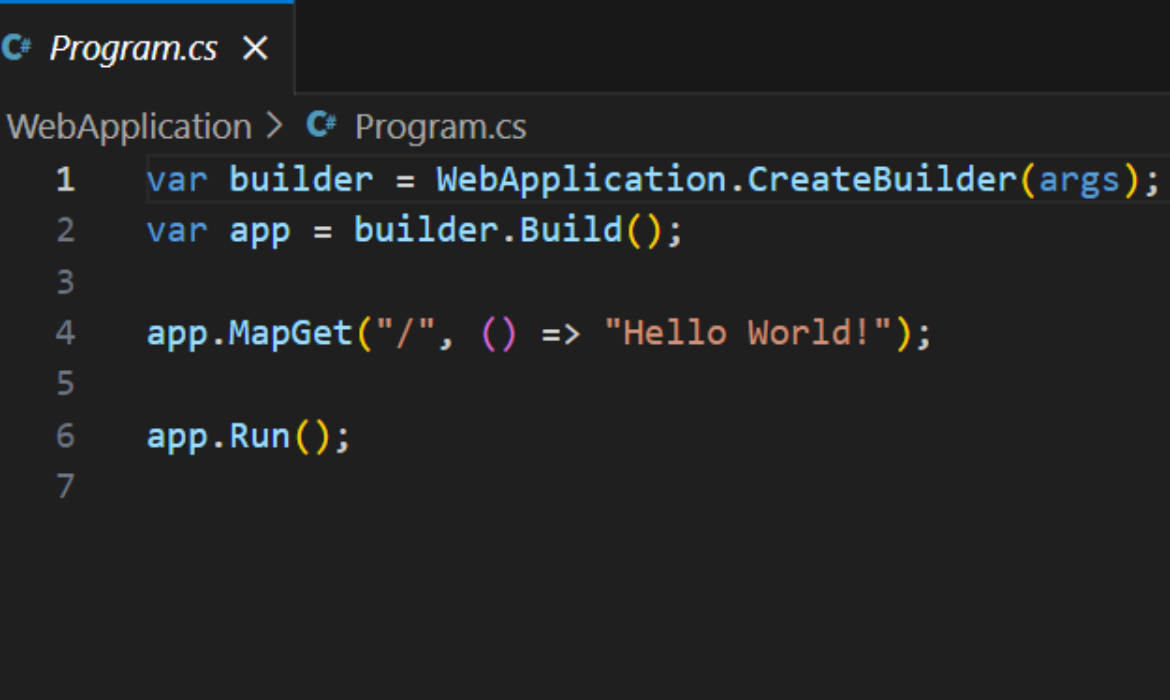
Introduction
As a .NET developer, you will often handle various file operations, such as reading from or writing to files. One common error you might encounter is the FileNotFoundException
. This error occurs when your program attempts to access a file that does not exist. Understanding the causes of this exception and knowing how to resolve it are crucial for building robust applications. In this blog post, we’ll delve into the details of FileNotFoundException
, its common causes, and how to effectively handle it.
What is FileNotFoundException?
FileNotFoundException
is an exception thrown by the .NET framework when an attempt to access a file that does not exist fails. This can happen during various file operations, such as opening a file for reading or writing.
Common Causes of FileNotFoundException
- Incorrect File Path: The most common cause of
FileNotFoundException
is an incorrect file path. This could be due to typographical errors, wrong directory paths, or the file not being in the expected location. - File Deleted or Moved: The file might have been deleted or moved to a different location after the path was specified in the code.
- Case Sensitivity: On systems that are case-sensitive, like Unix-based systems, mismatches in the case of the file name can lead to this exception.
- Permissions Issues: Lack of proper permissions to access the file can also result in a
FileNotFoundException
, although this is less common and often throws different exceptions.
How to Handle FileNotFoundException
- Verify File Path: Ensure that the file path specified in the code is correct and the file exists at that location. This can be done using tools or writing a simple check in your code.csharpCopy code
if (!File.Exists(filePath)) { Console.WriteLine("File not found: " + filePath); return; }
- Use Try-Catch Block: Wrap your file access code in a try-catch block to gracefully handle the exception and provide meaningful error messages.csharpCopy code
try { using (StreamReader sr = new StreamReader(filePath)) { // Read file contents } } catch (FileNotFoundException ex) { Console.WriteLine("File not found: " + ex.FileName); }
- Logging and Alerts: Implement logging to record such errors, which can be useful for debugging and alerting purposes.csharpCopy code
try { // File operations } catch (FileNotFoundException ex) { Logger.Log("File not found: " + ex.FileName); AlertService.NotifyAdmin("Critical file missing: " + ex.FileName); }
- Ensure Deployment: Make sure that all necessary files are included in your deployment package and are deployed to the correct locations.
Preventing FileNotFoundException
- Input Validation: Validate all input paths to ensure they are correct and point to existing files.
- Environment Configuration: Use environment variables or configuration files to manage file paths, making it easier to adjust paths without changing the code.
- Automated Tests: Write automated tests that include checks for file existence, especially for critical files that your application depends on.
- Proper Exception Handling: Always include robust exception handling for file operations to ensure your application can handle missing files gracefully.
Conclusion
The FileNotFoundException
is a common but easily manageable exception in .NET development. By understanding its causes and implementing proper checks and exception handling, you can prevent and resolve this error efficiently. Ensuring accurate file paths, validating inputs, and using try-catch blocks are some of the best practices to handle FileNotFoundException
. By following these guidelines, you can enhance the robustness and reliability of your applications.